Update #2
This week, I created json structure for terrain generation, and completed the Build function which takes the PGS json file and ouputs binary mesh files for rendering.
​
JSON file format:
In JSON file structure specifies following parameters:

x_border and y_border:
- These borders serve as the spatial limits within which the terrain mesh is generated.
step:
- The step size determines the resolution or spacing between points on the terrain grid.
- A smaller step value increases the density of points, resulting in finer terrain detail, while a larger step size reduces detail but improves rendering performance.
seed:
- The seed parameter influences terrain randomness.
- A negative seed (-1 in this example) makes the system use a time-based or dynamic value, giving a new terrain variation with each build.
- Setting a fixed seed instead would ensure the same terrain is generated each time, which is useful for testing and consistency.
- The seed will be output every build, so that users can refer to it to generate consistent terrain.
scale:
- The scale controls the overall “zoom” level of the terrain features.
- Higher scale values will stretch the terrain, creating broader, less frequent features, while lower values bring features closer together.
lacunarity:
- Lacunarity influences the frequency of each octave in the fractal noise.
- Lacunarity values below 1.0 reduce frequency with each octave, creating smoother terrain transitions between octaves, while values above 1.0 increase frequency and make the terrain more complex.
gain:
- Gain controls the amplitude of each octave in the fractal noise, affecting terrain roughness.
- Higher gain values amplify the influence of higher-frequency octaves, creating sharper, more pronounced terrain variations.
octaves:
- This parameter specifies the number of noise layers, used to add detail to the terrain.
- Each octave adds finer detail, with higher values resulting in more complex terrain. However, too many octaves can make the terrain look overly noisy and affect performance.
height_range:
- This range restricts the terrain elevation. Generated noise value will be normalized into this range.
Noise Value generation:
Using stb_perlin.h, incorporating parameters declared above, a 2D vector of float is generated.
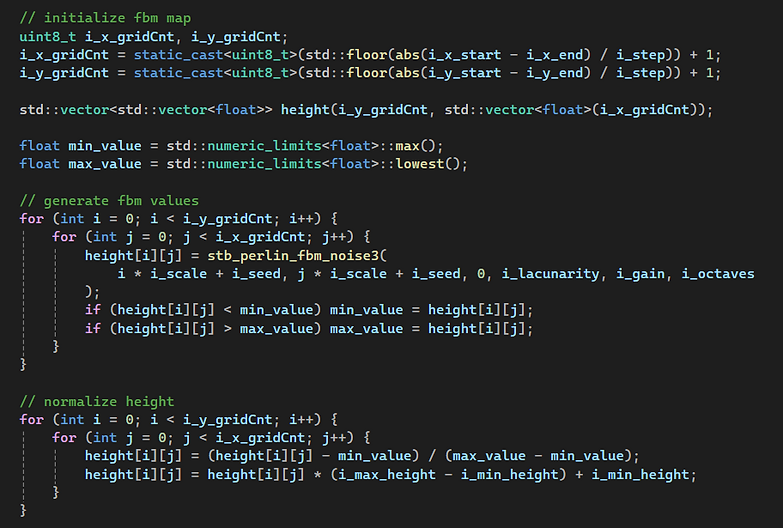
Generate mesh files:
In previous step, a 2D vector of noise value is generated. To make it into a triangle based mesh file, we can first consider the 2D vector made up of multiple squares. For one square, there are two triangles.
For vertice data, the system outputs the float in the original 2D vector order. For the indice data, the system iterate through each sqaure and outputs the indice order as triangles.​

Mesh file indice limit challenge:
I encountered a significant challenge of the limit of mesh files. In the previous work, the mesh files are limited to have at most 2^16 vertice and 2^16 indice. However, in terrain generation, the grid points easily exceed the limit.​
To solve the problem, I planned to separate the 2D array into pieces, while each piece holds < 2^16 indices and vertices. The count of vertices can be directly computed by row*column, but the count of indices are more complex. After computation, the indice count is (row-1)*(column-1)*6, which should be less than 2^16.
The separation algorithm needs to be efficient, which aims to generate the least binary files, because loading the binary files at runtime takes a great deal of time. I tried several ways including dynamic planning, but I found that the tasks needs to be done with limitation of grid dimensions. I researched into the topic and set the limit to be 2048*2048 temporally, and I aim to adjust it after testing.
With the limitation, the number of generated mesh files will be limited to 64. The separation will also be much easier. Since the dimensions are limited to 2048, the system will separate the vector by rows. For example, every 10 rows will be separated into one piece.
​
